Objectives
Object Oriented Technology is regarded as the ultimate paradigm for the modeling of information, be that information data or logic. The C+ + has by now shown to fulfill this goal.
Object-Oriented Technology (ऑब्जेक्ट-ओरिएंटेड टेक्नोलॉजी)
English:
Object-Oriented Technology is regarded as the ultimate paradigm for the modeling of information, whether that information is data or logic. It focuses on representing real-world objects and their interactions through objects and classes. Object-Oriented Programming (OOP) allows for more efficient management and organization of complex systems. C++ has been proven to fulfill this goal by incorporating essential object-oriented principles like inheritance, polymorphism, encapsulation, and abstraction.
Hindi:
ऑब्जेक्ट-ओरिएंटेड टेक्नोलॉजी को सूचना के मॉडलिंग के लिए अंतिम पैरेडाइम (दृष्टिकोण) माना जाता है, चाहे वह सूचना डेटा हो या लॉजिक। यह वास्तविक दुनिया की वस्तुओं और उनके इंटरएक्शन को ऑब्जेक्ट्स और क्लासेस के माध्यम से प्रदर्शित करने पर केंद्रित है। ऑब्जेक्ट-ओरिएंटेड प्रोग्रामिंग (OOP) जटिल प्रणालियों के प्रबंधन और संगठन को अधिक प्रभावी बनाता है। C++ ने इस लक्ष्य को पूरा करने के लिए आवश्यक ऑब्जेक्ट-ओरिएंटेड सिद्धांतों जैसे इनहेरिटेंस (विरासत), पॉलिमॉर्फिज्म (बहुरूपता), एन्कैप्सुलेशन (संवरण), और एब्सट्रैक्शन (सारनीकरण) को शामिल किया है।
Introduction To C++
C+ + character set, C+ + Tokens (Identifiers, Keywords, Constants, Operators) Structure of a C+ + Program (include files, declaration of an object , main function) Header files iostream.h, iomanip.h cout, cin. Use of I /O operators (<< and >>), Use of setw( ) and endl, Cascading of I /O operators, Error Message Use of editor, basic commands of editor, compilation, linking and execution.
Data Types, Variables and Constants
Concept of Data types Built – in Data types void, char, int, float and double Constants Integer Constants, Character Constants (Backslash character constants: \n \a, \r, \t), Floating point Constants, St ring Constants Variables of built – in data types, Access Modifier const type modifiers signed, unsigned, short, long.
Notes on C++ (English and Hindi)
1. C++ Character Set (C++ कैरेक्टर सेट)
English:
The character set in C++ includes all the characters that are used to write a C++ program. These characters are:
- Letters: Uppercase (A-Z) and lowercase (a-z) alphabets.
- Digits: 0-9.
- Special characters: Punctuation marks like
!, @, #, $, %, ^, &, *, (, )
. - Whitespace characters: Space, tab, newline.
- Escape sequences: Like
\n
(new line),\t
(tab),\\
(backslash).
Hindi:
C++ में कैरेक्टर सेट उन सभी कैरेक्टरों को शामिल करता है जिन्हें C++ प्रोग्राम लिखने के लिए उपयोग किया जाता है। ये कैरेक्टर निम्नलिखित होते हैं:
- अक्षर: अपरकेस (A-Z) और लोअरकेस (a-z) अक्षर।
- अंकों: 0-9।
- विशेष प्रतीक: विराम चिह्न जैसे
!, @, #, $, %, ^, &, *, (, )
। - व्हाइटस्पेस कैरेक्टर: स्पेस, टैब, न्यूलाइन।
- एस्केप सीक्वेंस: जैसे
\n
(नया लाइन),\t
(टैब),\\
(बैकस्लैश)।
2. C++ Tokens (C++ टोकन)
English:
Tokens are the smallest units in a C++ program. They include:
- Identifiers:
Names given to variables, functions, classes, etc. Examples:int a;
,void func()
. - Keywords:
Reserved words that have a special meaning in C++. Examples:int
,float
,if
,else
,for
,while
. - Constants:
Fixed values used in a program. Examples:100
,3.14
,"Hello"
. - Operators:
Symbols used to perform operations on variables and data. Examples:+
,-
,*
,/
,=
,++
,--
.
Hindi:
C++ में टोकन प्रोग्राम की सबसे छोटी इकाइयाँ होती हैं। इनमें शामिल हैं:
- पहचानकर्ता (Identifiers):
वेरिएबल्स, फंक्शंस, क्लासेस आदि को दिए गए नाम। उदाहरण:int a;
,void func()
. - किवर्ड्स (Keywords):
आरक्षित शब्द जिनका C++ में विशेष अर्थ होता है। उदाहरण:int
,float
,if
,else
,for
,while
। - स्थिरांक (Constants):
प्रोग्राम में उपयोग की जाने वाली स्थिर मान। उदाहरण:100
,3.14
,"Hello"
। - ऑपरेटर्स (Operators):
वेरिएबल्स और डेटा पर ऑपरेशन्स करने के लिए उपयोग किए जाने वाले प्रतीक। उदाहरण:+
,-
,*
,/
,=
,++
,--
।
3. Structure of a C++ Program (C++ प्रोग्राम की संरचना)
English:
A C++ program typically consists of the following components:
- Include Files:
The program may include header files to use standard libraries, such as#include <iostream>
for input and output operations. - Declaration of an Object:
Declaring objects and variables that will be used in the program.
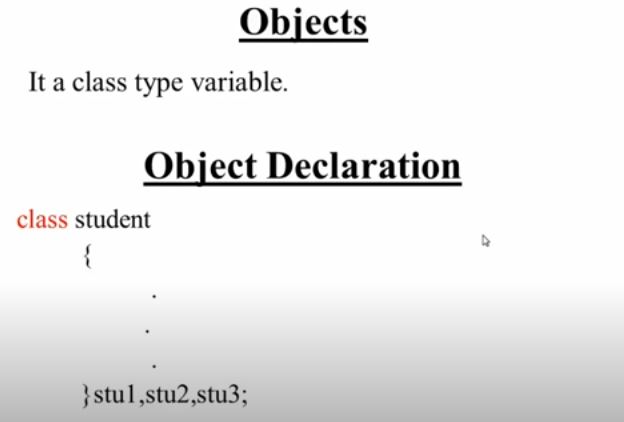
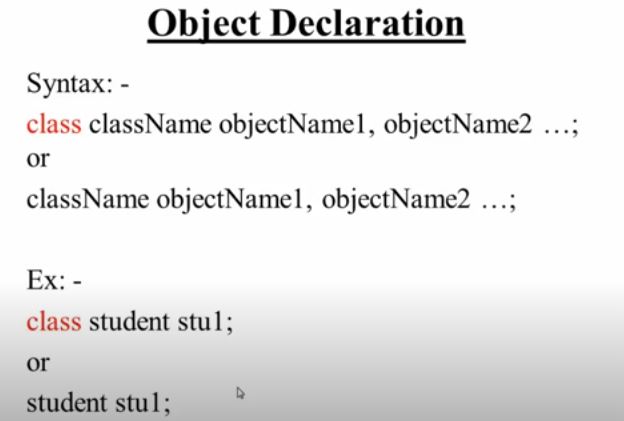
3. Main Function:
The entry point of the C++ program where the execution begins. It is written as:int main() { ... }
Hindi:
C++ प्रोग्राम सामान्यतः निम्नलिखित घटकों से बना होता है:
- इंक्लूड फाइल्स (Include Files):
प्रोग्राम में मानक लाइब्रेरी का उपयोग करने के लिए हेडर फाइल्स को शामिल किया जाता है, जैसे#include <iostream>
इनपुट और आउटपुट संचालन के लिए। - ऑब्जेक्ट की घोषणा (Declaration of an Object):
वे ऑब्जेक्ट्स और वेरिएबल्स जिन्हें प्रोग्राम में उपयोग किया जाएगा, उनकी घोषणा। - मुख्य फंक्शन (Main Function):
C++ प्रोग्राम का प्रवेश बिंदु जहाँ से निष्पादन शुरू होता है। यह इस प्रकार लिखा जाता है:int main() { ... }
4. Header Files (हेडर फाइल्स)
English:
- iostream.h:
It is used for input/output operations, allowing us to usecin
andcout
. - iomanip.h:
It is used for manipulating input/output format, e.g.,setw()
for setting the width of the output.
Hindi:
- iostream.h:
इसका उपयोग इनपुट/आउटपुट संचालन के लिए किया जाता है, जिससे हमcin
औरcout
का उपयोग कर सकते हैं। - iomanip.h:
इसका उपयोग इनपुट/आउटपुट के प्रारूप को संशोधित करने के लिए किया जाता है, जैसे आउटपुट की चौड़ाई सेट करने के लिएsetw()
।
5. Use of I/O Operators (I/O ऑपरेटर्स का उपयोग)
English:
- << (Insertion Operator):
Used withcout
to send data to the output stream. - >> (Extraction Operator):
Used withcin
to receive input from the user.
Hindi:
- << (इन्सर्शन ऑपरेटर):
इसका उपयोगcout
के साथ आउटपुट स्ट्रीम में डेटा भेजने के लिए किया जाता है। - >> (एक्सट्रैक्शन ऑपरेटर):
इसका उपयोगcin
के साथ उपयोगकर्ता से इनपुट प्राप्त करने के लिए किया जाता है।
6. Use of setw() and endl (setw() और endl का उपयोग)
English:
- setw():
It is used to set the width of the output for proper alignment. - endl:
It is used to insert a newline in the output and flush the output buffer.
Hindi:
- setw():
इसका उपयोग आउटपुट को सही तरीके से संरेखित करने के लिए आउटपुट की चौड़ाई सेट करने के लिए किया जाता है। - endl:
इसका उपयोग आउटपुट में एक नई पंक्ति जोड़ने और आउटपुट बफर को फ्लश करने के लिए किया जाता है।
7. Cascading of I/O Operators (I/O ऑपरेटर्स का कैस्केडिंग)
English:
Cascading means using multiple I/O operators together in a single statement. Example:cout << "Hello" << " " << "World" << endl;
Hindi:
कैस्केडिंग का मतलब है एक ही स्टेटमेंट में एक साथ कई I/O ऑपरेटर्स का उपयोग करना। उदाहरण:cout << "Hello" << " " << "World" << endl;
8. Error Message (एरर संदेश)
English:
Error messages are shown during the compilation process when the program contains errors. These messages provide information about the type of error and its location in the program.
Hindi:
एरर संदेश वे संदेश होते हैं जो संकलन प्रक्रिया के दौरान तब दिखाई देते हैं जब प्रोग्राम में कोई त्रुटि होती है। ये संदेश त्रुटि के प्रकार और उसके स्थान के बारे में जानकारी प्रदान करते हैं।
9. Use of Editor, Basic Commands of Editor, Compilation, Linking, and Execution (एडिटर का उपयोग, एडिटर के बुनियादी आदेश, संकलन, लिंकिंग और निष्पादन)
English:
- Editor:
It is a software used for writing and editing code, such as Notepad++, Sublime Text, or Visual Studio Code. - Basic Commands of Editor:
Commands like Save (Ctrl + S
), Open (Ctrl + O
), Compile, Run. - Compilation:
The process of translating the source code into machine code using a compiler. - Linking:
The process of linking the object files and libraries to create the executable file. - Execution:
Running the compiled program to produce the desired output.
Hindi:
- एडिटर (Editor):
यह एक सॉफ़्टवेयर होता है जिसका उपयोग कोड लिखने और संपादित करने के लिए किया जाता है, जैसे Notepad++, Sublime Text, या Visual Studio Code। - एडिटर के बुनियादी आदेश (Basic Commands of Editor):
आदेश जैसे Save (Ctrl + S
), Open (Ctrl + O
), Compile, Run। - संकलन (Compilation):
यह प्रक्रिया स्रोत कोड को मशीन कोड में बदलने के लिए संकलक (compiler) का उपयोग करती है। - लिंकिंग (Linking):
यह प्रक्रिया ऑब्जेक्ट फाइल्स और लाइब्रेरीज़ को जोड़ने की प्रक्रिया होती है ताकि एक कार्यशील फ़ाइल बनाई जा सके। - निष्पादन (Execution):
संकलित प्रोग्राम को चलाना ताकि इच्छित आउटपुट प्राप्त किया जा सके।
These notes cover the basic concepts and structure of C++ Programming in both English and Hindi. Let me know if you need any further details!
Operators and Expressions
Operators: Arithmetic operators ( – , + , / , % ) , Urinary operator ( – ), Increment and Decrement Operators ( – – , + + ) , Relational Operators.(> ,> = , < ,< = ,= != ), Logical operators (&&,I I , ! ), conditional operator ?: ( condition ? if statements: else-statements) , Precedence of Operators Expressions Automatic Type conversion in expressions, C+ + shorthands (+ = , -= , = , / = , %= ) Assignment statement : Variable initializations Type Compatibility, Type casting.
Flow of Control
Conditional Statements: if-else statement if-else- if- ladder, nested if,? As alternative to if, switch-case, Nested switch, default statement, break statement Simple Control statement, Comma Operator, exit 0 function Loops while statement, do-while statement, for statement, nested control structures.
Programming Methodology
General Concepts Modular approach Stylistic Guidelines Clarity and Simplicity of Expressions, Names, Comments, Indentation Documentation and Program Maintenance Running and Debugging programs, Syntax Errors, Run-Time Errors, Logic Errors.
Input Output Functions
Header file: sdtio.h – getc( ), putc( ), gets( ) and puts( ) functions.
Built-in Function
Header files: string.h, ctype.h, math.h, stdIib.h. Standard functions – character and st ring related functions: isalnumO, isalpha( ), isdigit ( ), islower( ), isupper( ), tolower, toupper( ), st rcpy( ), st rcat ( ), st rien( ), st rcmp( ) st rcmpi( ), atoi( ), itoa( ), ato1( ), 1toa( ) Mathemat ical funct ions: abs( ), sqrt ( ), exp( ), log( ), log10( ), frexp( ), sin( ), cos( ).
Functions
Defining a function Function Prototype, Invoking a function Passing arguments to function, specifying argument data types, default arguments, constant arguments, call by value, call by reference, returning values from a function, calling functions with arrays scope rules of functions and variables.
Structured Data Type: Array
Use of arrays one and two dimensional arrays – declaration, initialisation, reading, display, manipulation. such as liner search, finding maximum/minimum value, matrix arithmetic String: St ring manipulations such as reversing a string, reversing each word of a string, counting vowels, consonants, special characters from string.